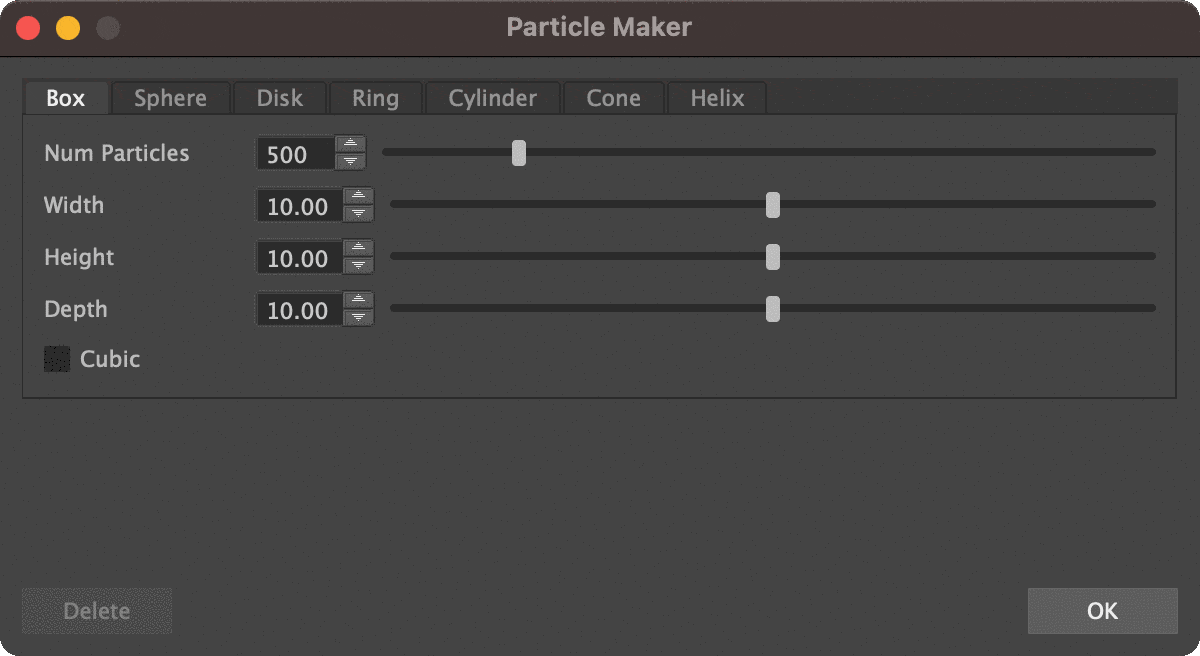
Abstract
Using Object-Oriented Programming concepts, Python and PySide, develop a script that
- Creates a user interface with parameters’ sliders and spinboxes to create nParticles of different geometric shapes in Autodesk Maya.
- Also, make use of tabbed pages and checkboxes to make your interface more intuitive.
- Finally, assign optimal default values to each function.
Object-Oriented Programming
OOP has always been a scary concept ever since I gotten into coding and programming. It no doubt is a difficult subject to learn, so that is why I will enhance my learning by teaching OOP in the early part of this study.
- Why use objects? It allow us to acquire logically group our data and functions that we can easily reuse or build upon. To create an object, we need to 1. declare with a class followed bt its name and parenthesis, and initialize it with a def__init__.
- What is the init function for? Constructors are used to initialize the object’s state. The task of constructors is to initialize(assign values) to the data members of the class when an object of class is created. Like methods, a constructor also contains collection of statements(i.e. instructions) that are executed at time of Object creation. It is run as soon as an object of a class is instantiated. The method is useful to do any initialization you want to do with your object.
- What is a good mental model to think about Class? Think of a class as a series of functions combined with variables, a framework to keep attributes and methods consistent a blueprint to feed in data and access the data.
Putting object into a class
In Professor Kesson’s introduction to OOP, he made an example using vertebrate and pets. What confuses me at first is the putting a class into another class. Doing so allows a class to inherit all methods and attributes from inputted class. So in the bottom example,
- dog = vertibrate(‘canine’) = create a object named “dog” and this inherit all the methods eg. get_mammal(), set_mammal(), add_mammal.
- The object is initialize with __init__(‘canine’)* and the self.mammal = ‘canine’. Notice how the there is no need to input into self parameter.
- print(dog.get_mammal()) = access vertebrate method of get_mammal which retrieve the initialize data we got from above.
User Interface Overview
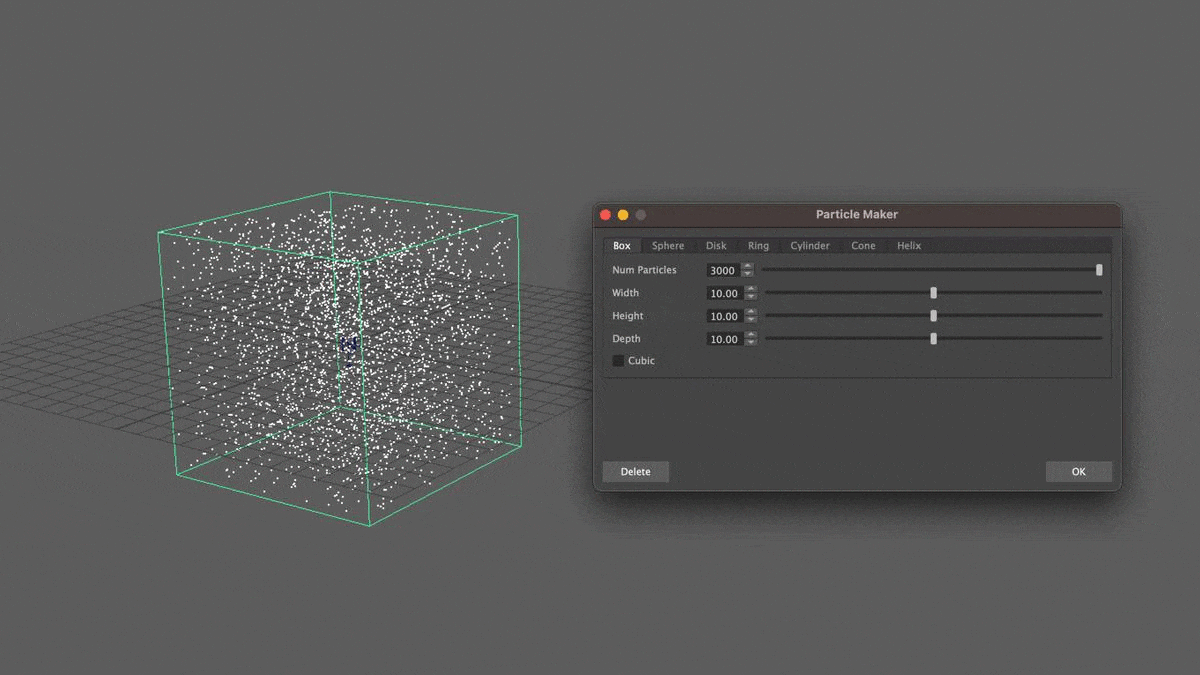
Enhancing the user experience
Instead of deleting the nParticles from the Outliner, a “Delete” button is added that removes the last nParticle object created. It also makes use of conditional statement to disable the button when there is no more nParticle. This will prevent users from being tempted to click the button and result in error if any.
In addition, with help of Professor’s guidance, I also added a checkbox that allows one to create a cube from the Box Tab page instead of having a separate Tab page. By checking the checkbox, it disables the the height and depth parameter, and only uses the width for all sides of a cube.
User Instructions
INSTALLATION
- Download the script below
- Place the scripts in your Maya script directory: ~/Documents/maya/scripts/
HOW TO LAUNCH
- In Maya, open up Script Editor
- Run the following code to use the User Interface
Conclusion
As much as the assignment was to make the UI, I feel that the main takeaway is writing modules for different UI elements such as the tab pages, sliders or spinboxes, and importing them into the main module as classes or objects to use to do whatever with want. My understanding of Object-Oriented Programming has definitely improved and I feel more confident implementing it in Python.